1.1: Create Your First Android App
Contents:
- The development process
- Using Android Studio
- Exploring a project
- Viewing and editing Java code
- Viewing and editing layouts
- Understanding the build process
- Running the app on an emulator or a device
- Using the log
- Related practical
- Learn more
This chapter describes how to develop applications using the Android Studio Integrated Development Environment (IDE).
The development process
An Android app project begins with an idea and a definition of the requirements necessary to realize that idea. As the project progresses, it goes through design, development, and testing.
The above diagram is a high-level picture of the development process, with the following steps:
- Defining the idea and its requirements: Most apps start with an idea of what it should do, bolstered by market and user research. During this stage the app's requirements are defined.
- Prototyping the user interface: Use drawings, mock ups and prototypes to show what the user interface would look like, and how it would work.
- Developing and testing the app: An app consists of one or more activities. For each activity you can use Android Studio to do the following, in no particular order:
- Create the layout: Place UI elements on the screen in a layout, and assign string resources and menu items, using the Extensible Markup Language (XML).
- Write the Java code: Create source code for components and tests, and use testing and debugging tools.
- Register the activity: Declare the activity in the manifest file.
- Define the build: Use the default build configuration or create custom builds for different versions of your app.
- Publishing the app: Assemble the final APK (package file) and distribute it through channels such as the Google Play.
Using Android Studio
Android Studio provides tools for the testing, and publishing phases of the development process, and a unified development environment for creating apps for all Android devices. The development environment includes code templates with sample code for common app features, extensive testing tools and frameworks, and a flexible build system.
Starting an Android Studio project
After you have successfully installed the Android Studio IDE, double-click the Android Studio application icon to start it. Choose Start a new Android Studio project in the Welcome window, and name the project the same name that you want to use for the app.
When choosing a unique Company Domain, keep in mind that apps published to the Google Play must have a unique package name. Since domains are unique, prepending the app's name with your name, or your company's domain name, should provide an adequately unique package name. If you are not planning to publish the app, you can accept the default example domain. Be aware that changing the package name later is extra work.
Choosing target devices and the minimum SDK
When choosing Target Android Devices, Phone and Tablet are selected by default, as shown in the figure below. The choice shown in the figure for the Minimum SDK — API 15: Android 4.0.3 (IceCreamSandwich) — makes your app compatible with 97% of Android devices active on the Google Play Store.
Different devices run different versions of the Android system, such as Android 4.0.3 or Android 4.4. Each successive version often adds new APIs not available in the previous version. To indicate which set of APIs are available, each version specifies an API level. For instance, Android 1.0 is API level 1 and Android 4.0.3 is API level 15.
The Minimum SDK declares the minimum Android version for your app. Each successive version of Android provides compatibility for apps that were built using the APIs from previous versions, so your app should always be compatible with future versions of Android while using the documented Android APIs.
Choosing a template
Android Studio pre-populates your project with minimal code for an activity and a screen layout based on a template. A variety of templates are available, ranging from a virtually blank template (Add No Activity) to various types of activities.
You can customize the activity after choosing your template. For example, the Empty Activity template provides a single activity accompanied by a single layout resource for the screen. You can choose to accept the commonly used name for the activity (such as MainActivity) or change the name on the Customize the Activity screen. Also, if you use the Empty Activity template, be sure to check the following if they are not already checked:
- Generate Layout file: Leave this checked to create the layout resource connected to this activity, which is usually named activity_main.xml. The layout defines the user interface for the activity.
- Backwards Compatibility (AppCompat): Leave this checked to include the AppCompat library so that the app is compatible with previous versions of Android even if it uses features found only in newer versions.
Android Studio creates a folder for the newly created project in the AndroidStudioProjects folder on your computer.
Android Studio window panes
The Android Studio main window is made up of several logical areas, or panes, as shown in the figure below.
In the above figure:
- The Toolbar.The toolbar carries out a wide range of actions, including running the Android app and launching Android tools.
- The Navigation Bar. The navigation bar allows navigation through the project and open files for editing. It provides a more compact view of the project structure.
- The Editor Pane. This pane shows the contents of a selected file in the project. For example, after selecting a layout (as shown in the figure), this pane shows the layout editor with tools to edit the layout. After selecting a Java code file, this pane shows the code with tools for editing the code.
- The Status Bar. The status bar displays the status of the project and Android Studio itself, as well as any warnings or messages. You can watch the build progress in the status bar.
- The Project Pane. The project pane shows the project files and project hierarchy.
- The Monitor Pane. The monitor pane offers access to the TODO list for managing tasks, the Android Monitor for monitoring app execution (shown in the figure), the logcat for viewing log messages, and the Terminal application for performing Terminal activities.
Tip: You can organize the main window to give yourself more screen space by hiding or moving panes. You can also use keyboard shortcuts to access most features. See Keyboard Shortcuts for a complete list.
Exploring a project
Each project in Android Studio contains the AndroidManifest.xml file, component source-code files, and associated resource files. By default, Android Studio organizes your project files based on the file type, and displays them within the Project: Android view in the left tool pane, as shown below. The view provides quick access to your project's key files.
To switch back to this view from another view, click the vertical Project tab in the far left column of the Project pane, and choose Android from the pop-up menu at the top of the Project pane, as shown in the figure below.
In the figure above:
- The Project tab. Click to show the project view.
- The Android selection in the project drop-down menu.
- The AndroidManifest.xml file. Used for specifying information about the app for the Android runtime environment. The template you choose creates this file.
- The java folder. This folder includes activities, tests, and other components in Java source code. Every activity, service, and other component is defined as a Java class, usually in its own file. The name of the first activity (screen) the user sees, which also initializes app-wide resources, is customarily MainActivity.
- The res folder. This folder holds resources, such as XML layouts, UI strings, and images. An activity usually is associated with an XML resource file that specifies the layout of its views. This file is usually named after its activity or function.
- The build.gradle (Module: App) file. This file specifies the module's build configuration. The template you choose creates this file, which defines the build configuration, including the
minSdkVersion
attribute that declares the minimum version for the app, and thetargetSdkVersion
attribute that declares the highest (newest) version for which the app has been optimized. This file also includes a list of dependencies, which are libraries required by the code — such as the AppCompat library for supporting a wide range of Android versions.
1
Viewing the Android Manifest
Before the Android system can start an app component, the system must know that the component exists by reading the app's AndroidManifest.xml file. The app must declare all its components in this file, which must be at the root of the app project directory.
To view this file, expand the manifests folder in the Project: Android view, and double-click the file (AndroidManifest.xml). Its contents appear in the editing pane as shown in the figure below.
Android namespace and application tag
The Android Manifest is coded in XML and always uses the Android namespace:
xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.android.helloworld">
The package
expression shows the unique package name of the new app. Do not change this once the app is published.
<application
...
</application>
The <application
tag, with its closing </application>
tag, defines the manifest settings for the entire app.
Automatic backup
The android:allowBackup
attribute enables automatic app data backup:
...
android:allowBackup="true"
...
Setting the android:allowBackup
attribute to true
enables the app to be backed up automatically and restored as needed. Users invest time and effort to configure apps. Switching to a new device can cancel out all that careful configuration. The system performs this automatic backup for nearly all app data by default, and does so without the developer having to write any additional app code.
For apps whose target SDK version is Android 6.0 (API level 23) and higher, devices running Android 6.0 and higher automatically create backups of app data to the cloud because the android:allowBackup
attribute defaults to true
if omitted. For apps < API level 22 you have to explicitly add the android:allowBackup
attribute and set it to true
.
Tip: To learn more about the automatic backup for apps, see Configuring Auto Backup for Apps.
The app icon
The android:icon
attribute sets the icon for the app:
...
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
...
The android:icon
attribute assigns an icon in the mipmap folder (inside the res folder in Project: Android view) to the app. The icon appears in the Launcher for launching the app. The icon is also used as the default icon for app components.
App label and string resources
As you can see in the previous figure, the android:label
attribute shows the string "Hello World"
highlighted. If you click on this string, it changes to show the string resource @string/app_name
:
...
android:label="@string/app_name"
...
Tip: Ctrl-click or right-click app_name
in the edit pane to see the context menu. Choose Go To > Declaration to see where the string resource is declared: in the strings.xml file. When you choose Go To > Declaration or open the file by double-clicking strings.xml in the Project: Android view (inside the values folder), its contents appear in the editing pane.
After opening the strings.xml file, you can see that the string name app_name
is set to Hello World
. You can change the app name by changing the Hello World
string to something else. String resources are described in a separate lesson.
The app theme
The android:theme
attribute sets the app's theme, which defines the appearance of user interface elements such as text:
...
android:theme="@style/AppTheme">
...
The theme
attribute is set to the standard theme AppTheme
. Themes are described in a separate lesson.
Declaring the Android version
Different devices may run different versions of the Android system, such as Android 4.0 or Android 4.4. Each successive version can add new APIs not available in the previous version. To indicate which set of APIs are available, each version specifies an API level. For instance, Android 1.0 is API level 1 and Android 4.4 is API level 19.
The API level allows a developer to declare the minimum version with which the app is compatible, using the <uses-sdk>
manifest tag and its minSdkVersion
attribute. For example, the Calendar Provider APIs were added in Android 4.0 (API level 14). If your app can't function without these APIs, declare API level 14 as the app's minimum supported version like this:
<manifest ... >
<uses-sdk android:minSdkVersion="14" android:targetSdkVersion="19" />
...
</manifest>
The minSdkVersion
attribute declares the minimum version for the app, and the targetSdkVersion
attribute declares the highest (newest) version which has been optimized within the app. Each successive version of Android provides compatibility for apps that were built using the APIs from previous versions, so the app should always be compatible with future versions of Android while using the documented Android APIs.
The targetSdkVersion
attribute does not prevent an app from being installed on Android versions that are higher (newer) than the specified value, but it is important because it indicates to the system whether the app should inherit behavior changes in newer versions. If you don't update the targetSdkVersion
to the latest version, the system assumes that your app requires some backward-compatibility behaviors when running on the latest version. For example, among the behavior changes in Android 4.4, alarms created with the AlarmManager APIs are now inexact by default so that the system can batch app alarms and preserve system power, but the system will retain the previous API behavior for an app if your target API level is lower than "19"
.
Viewing and editing Java code
Components are written in Java and listed within module folders in the java folder in the Project: Android view. Each module name begins with the domain name (such as com.example.android) and includes the app name.
The following example shows an activity component:
- Click the module folder to expand it and show the MainActivity file for the activity written in Java (the
MainActivity
class). - Double-click MainActivity to see the source file in the editing pane, as shown in the figure below.
At the very top of the MainActivity.java file is a package
statement that defines the app package. This is followed by an import
block condensed in the above figure, with "...
". Click the dots to expand the block to view it. The import
statements import libraries needed for the app, such as the following, which imports the AppCompatActivity library:
import android.support.v7.app.AppCompatActivity;
Each activity in an app is implemented as a Java class. The following class declaration extends the AppCompatActivity
class to implement features in a way that is backward-compatible with previous versions of Android:
public class MainActivity extends AppCompatActivity {
...
}
As you learned earlier, before the Android system can start an app component such as an activity, the system must know that the activity exists by reading the app's AndroidManifest.xml file. Therefore, each activity must be listed in the AndroidManifest.xml file.
Viewing and editing layouts
Layout resources are written in XML and listed within the layout folder in the res folder in the Project: Android view. Click res > layout and then double-click activity_main.xml to see the layout file in the editing pane.
Android Studio shows the Design view of the layout, as shown in the figure below. This view provides a Palette pane of user interface elements, and a grid showing the screen layout.
Understanding the build process
The Android application package (APK) is the package file format for distributing and installing Android mobile apps. The build process involves tools and processes that automatically convert each project into an APK.
Android Studio uses Gradle as the foundation of the build system, with more Android-specific capabilities provided by the Android Plugin for Gradle. This build system runs as an integrated tool from the Android Studio menu.
Understanding build.gradle files
When you create a project, Android Studio automatically generates the necessary build files in the Gradle Scripts folder in Project: Android view. Android Studio build files are named build.gradle as shown below:
Each project has the following:
build.gradle (Project: apptitle)
This is the top-level build file for the entire project, located in the root project directory, which defines build configurations that apply to all modules in your project. This file, generated by Android Studio, should not be edited to include app dependencies.
build.gradle (Module: app)
Android Studio creates separate build.gradle (Module: app)
files for each module. You can edit the build settings to provide custom packaging options for each module, such as additional build types and product flavors, and to override settings in the manifest or top-level build.gradle file. This file is most often the file to edit when changing app-level configurations, such as declaring dependencies in the dependencies
section. The following shows the contents of a project's build.gradle (Module: app)
file:
apply plugin: 'com.android.application'
android {
compileSdkVersion 24
buildToolsVersion "24.0.1"
defaultConfig {
applicationId "com.example.android.helloworld2"
minSdkVersion 15
targetSdkVersion 24
versionCode 1
versionName "1.0"
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', {
exclude group: 'com.android.support', module: 'support-annotations'
})
compile 'com.android.support:appcompat-v7:24.2.1'
testCompile 'junit:junit:4.12'
}
The build.gradle files use Gradle syntax. Gradle is a Domain Specific Language (DSL) for describing and manipulating the build logic using Groovy, which is a dynamic language for the Java Virtual Machine (JVM). You don't need to learn Groovy to make changes, because the Android Plugin for Gradle introduces most of the DSL elements you need.
Tip: To learn more about the Android plugin DSL, read the DSL reference documentation.
Plugin and Android blocks
In the above build.gradle (Module: app)
file, the first statement applies the Android-specific Gradle plug-in build tasks:
apply plugin: 'com.android.application'
android {
...
}
The android { }
block specifies the following for the build:
- The target SDK version for compiling the code:
compileSdkVersion 24
- The version of the build tools to use for building the app:
buildToolsVersion "24.0.1"
The defaultConfig block
Core settings and entries for the app are specified in a defaultConfig { }
block within the android { } block:
...
defaultConfig {
applicationId "com.example.hello.helloworld"
minSdkVersion 15
targetSdkVersion 23
versionCode 1
versionName "1.0"
testInstrumentationRunner
"android.support.test.runner.AndroidJUnitRunner"
}
...
The minSdkVersion
and targetSdkVersion
settings override any AndroidManifest.xml settings for the minimum SDK version and the target SDK version. See "Declaring the Android version" previously in this chapter for background information on these settings.
The testInstrumentationRunner
statement adds the instrumentation support for testing the user interface with Espresso and UIAutomator. These are described in a separate lesson.
Build types
Build types for the app are specified in a buildTypes { }
block, which controls how the app is built and packaged.
...
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'),
'proguard-rules.pro'
}
}
...
The build type specified is release
for the app's release. Another common build type is debug
. Configuring build types is described in a separate lesson.
Dependencies
Dependencies for the app are defined in the dependencies { }
block, which is the part of the build.gradle file that is most likely to change as you start developing code that depends on other libraries. The block is part of the standard Gradle API and belongs outside the android { }
block.
...
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', {
exclude group: 'com.android.support', module: 'support-annotations'
})
compile 'com.android.support:appcompat-v7:24.2.0'
testCompile 'junit:junit:4.12'
}
In the above snippet, the statement compile fileTree(dir: 'libs', include: ['*.jar'])
adds a dependency of all ".jar" files inside the libs
directory. The compile
configuration compiles the main application — everything in it is added to the compilation classpath, and also packaged into the final APK.
Syncing your project
When you make changes to the build configuration files in a project, Android Studio requires that you sync the project files so that it can import the build configuration changes and run some checks to make sure the configuration won't create build errors.
To sync the project files, click Sync Now in the notification bar that appears when making a change, or click Sync Project from the menu bar. If Android Studio notices any errors with the configuration — for example, if the source code uses API features that are only available in an API level higher than the compileSdkVersion
— the Messages window appears to describe the issue.
Running the app on an emulator or a device
With virtual device emulators, you can test an app on different devices such as tablets or smartphones — with different API levels for different Android versions — to make sure it looks good and works for most users. Although it's a good idea, you don't have to depend on having a physical device available for app development.
The Android Virtual Device (AVD) manager creates a virtual device or emulator that simulates the configuration for a particular type of Android device. Use the AVD Manager to define the hardware characteristics of a device and its API level, and to save it as a virtual device configuration. When you start the Android emulator, it reads a specified configuration and creates an emulated device on your computer that behaves exactly like a physical version of that device.
Creating a virtual device
To run an emulator on your computer, use the AVD Manager to create a configuration that describes the virtual device. Select Tools > Android > AVD Manager, or click the AVD Manager icon in the toolbar.
The "Your Virtual Devices" screen appears showing all of the virtual devices created previously. Click the +Create Virtual Device button to create a new virtual device.
You can select a device from a list of predefined hardware devices. For each device, the table shows its diagonal display size (Size), screen resolution in pixels (Resolution), and pixel density (Density). For example, the pixel density of the Nexus 5 device is xxhdpi
, which means the app uses the icons in the xxhdpi folder of the mipmap folder. Likewise, the app will use layouts and drawables from folders defined for that density as well.
You also choose the version of the Android system for the device. The Recommended tab shows the recommended systems for the device. More versions are available under the x86 Images and Other Images tabs.
Running the app on the virtual device
To run the app on the virtual device you created in the previous section, follow these steps:
- In Android Studio, select Run > Run app or click the Run icon
in the toolbar.
- In the Select Deployment Target window, under Available Emulators, select the virtual device you created, and click OK.
The emulator starts and boots just like a physical device. Depending on the speed of your computer, this may take a while. The app builds, and once the emulator is ready, Android Studio uploads the app to the emulator and runs it.
You should see the app created from the Empty Activity template ("Hello World") as shown in the following figure, which also shows Android Studio's Run pane that displays the actions performed to run the app on the emulator.
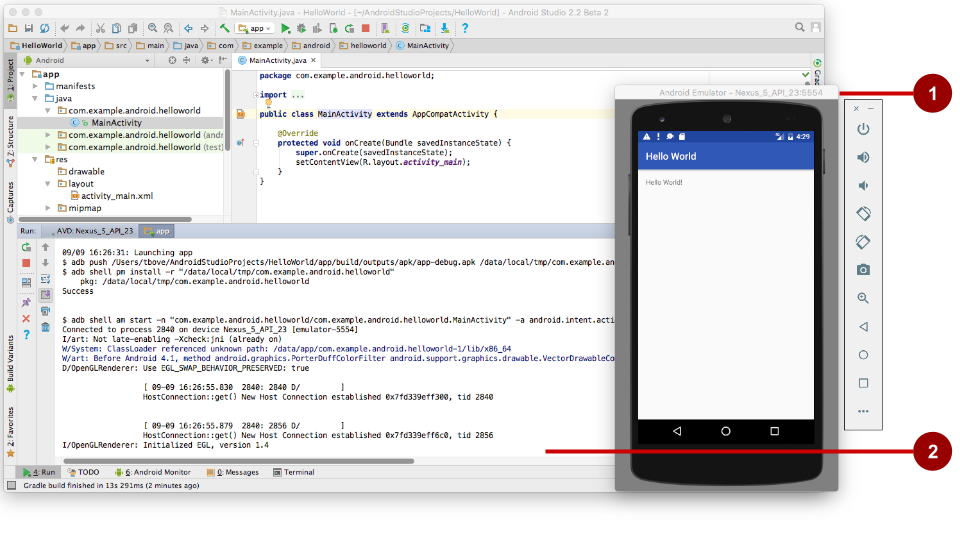
In the above figure:
- The Emulator running the app.
- The Run Pane. This shows the actions taken to install and run the app.
Running the app on a physical device
Always test your apps on physical device, because users will use the app on physical devices. While emulators are quite good, they can't show all possible device states, such as what happens if an incoming call occurs while the app is running. To run the app on a physical device, you need the following:
- An Android device such as a smartphone or tablet.
- A data cable to connect the Android device to your computer via the USB port.
- If you are using Linux or Windows, it may be necessary to perform additional steps to run the app on a hardware device. Check the Using Hardware Devices documentation. On Windows, you may need to install the appropriate USB driver for the device. See OEM USB Drivers.
To let Android Studio communicate with a device, turn on USB Debugging on the Android device. On Android version 4.2 and newer, the Developer options screen is hidden by default. Follow these steps to turn on USB Debugging:
- On the physical device, open Settings and choose About phone at the bottom of the Settings screen.
Tap the Build number information seven times.
You read that correctly: Tap it seven times.
- Return to the previous screen (Settings). Developer options now appears at the bottom of the screen. Tap Developer options.
- Choose USB Debugging.
Now, connect the device and run the app from Android Studio.
Troubleshooting the device connection
If Android Studio does not recognize the device, try the following:
- Disconnect the device from your computer, and then reconnect it.
- Restart Android Studio.
If your computer still does not find the device or declares it "unauthorized":
Disconnect the device from your computer.
On the device, choose Settings > Developer Options.
Tap Revoke USB Debugging authorizations.
Reconnect the device to your computer.
When prompted, grant authorizations.
- You may need to install the appropriate USB driver for the device. See Using Hardware Devices documentation.
- Check the latest documentation, programming forums, or get help from your instructors.
Using the log
The log is a powerful debugging tool you can use to look at values, execution paths, and exceptions. After you add logging statements to an app, your log messages appear along with general log messages in the logcat tab of the Android Monitor pane of Android Studio.
To see the Android Monitor pane, click the Android Monitor button at the bottom of the Android Studio main window. The Android Monitor offers two tabs:
- The logcat tab. The logcat tab displays log messages about the app as it is running. If you add logging statements to the app, your log messages from these statements appear with the other log messages under this tab.
- The Monitors tab. The Monitors tab monitors the performance of the app, which can be helpful for debugging and tuning your code.
Adding logging statements to your app
Logging statements add whatever messages you specify to the log. Adding logging statements at certain points in the code allows the developer to look at values, execution paths, and exceptions.
For example, the following logging statement adds "MainActivity"
and "Hello World"
to the log:
Log.d("MainActivity", "Hello World");
The following are the elements of this statement:
Log
: The Log class is the API for sending log messages.d
: You assign a log level so that you can filter the log messages using the drop-down menu in the center of the logcat tab pane. The following are log levels you can assign:d
: Choose Debug or Verbose to see these messages.e
: Choose Error or Verbose to see these messages.w
: Choose Warning or Verbose to see these messages.i
: Choose Info or Verbose to see these messages.
"MainActivity"
: The first argument is a log tag which can be used to filter messages under the logcat tab. This is commonly the name of the activity from which the message originates. However, you can make this anything that is useful to you for debugging the app. The best practice is to use a constant as a log tag, as follows:
- Define the log tag as a constant before using it in logging statement:
private static final String LOG_TAG = MainActivity.class.getSimpleName();
- Use the constant in the logging statements:
Log.d(LOG_TAG, "Hello World");
"Hello World"
: The second argument is the actual message that appears after the log level and log tag under the logcat tab.
Viewing your log messages
The Run pane appears in place of the Android Monitor pane when you run the app on an emulator or a device. After starting to run the app, click the Android Monitor button at the bottom of the main window, and then click the logcat tab in the Android Monitor pane if it is not already selected.
In the above figure:
- The logging statement in the
onCreate()
method ofMainActivity
. - Android Monitor pane showing
logcat
log messages, including the message from the logging statement.
By default, the log display is set to Verbose in the drop-down menu at the top of the logcat display to show all messages. You can change this to Debug to see messages that start with Log.d
, or change it to Error to see messages that start with Log.e
, and so on.
Related practical
The related exercises and practical documentation is in Android Developer Fundamentals: Practicals.
Learn more
- Android Studio documentation:
- Android API Guide, "Develop" section:
- Other:
- Android Studio User's Guide: Image Asset Studio
- Wikipedia: Summary of Android Version History
- Groovy syntax
- Gradle site